Introduction
Creating a seamless and efficient user experience is paramount to retaining customers and driving engagement. Did you know that a bad user experience can deter a whopping 88% of online shoppers from returning to a website? As a decision-maker, you understand the importance of structuring your web applications for both optimal performance and maintainability.
This blog post will guide you through the process of building a robust layout component in React, complete with a header and sidebar, using the latest version of React Router DOM. By implementing this structured approach, you can ensure a consistent and intuitive interface across your application, enhancing both user satisfaction and operational efficiency.
Whether you’re managing a development team or looking to improve your application’s architecture, this step-by-step guide will equip you with the knowledge to make informed decisions and elevate your digital presence.
Prerequisites
1. Basic Knowledge of React and React Router:
- Understanding of React: Familiarity with React fundamentals such as JSX, components, state, and props is crucial. If you’re new to React, consider going through the official React documentation or online tutorials.
- React Router Basics: A basic understanding of React Router is important since this guide uses React Router for routing. If you’re new to React Router, the React Router documentation is a great place to start.
- Tip: Try building a simple React application before attempting to implement complex routing. This step ensures you’re comfortable with React’s core concepts.
2. Node.js and npm/yarn Installed:
- js: Make sure you have Node.js installed on your system as it is required to run a React application. You can download it from the official Node.js website.
- Package Manager (npm/yarn): npm is automatically installed with Node.js. However, some developers prefer using yarn for its speed and reliability. You can choose either npm or yarn based on your preference.
- Installation Check: You can check if Node.js and npm are installed correctly by running node -v and npm -v in your terminal or command prompt.
- Tip: Keep your Node.js version up to date to avoid compatibility issues with newer packages.
3. A React Project Setup:
- Creating a React App: If you haven’t already, you can create a new React application using Create React App by running npx create-react-app my-app. Replace my-app with your project name.
- Project Structure Understanding: Familiarize yourself with the basic structure of a React project created with Create React App. This includes understanding where to place your components, routes, styles, etc.
- Tip: Organize your project structure from the beginning. Proper organization (like separating components, services, and utilities into different folders) makes your project easy to manage as it grows.
Transform Your Web Application Today!
Are you ready to elevate your web application's user experience with a robust and maintainable layout? Our team at AlphaBOLD is here to help you implement the best practices in React development, ensuring a seamless and intuitive interface for your users.
Request a ConsultationStep 1: Install React Router DOM
If you haven’t already installed React Router DOM, you can do so by running:
npm install react-router-dom
Step 2: Create the Layout Component
Our layout component will include a header and a sidebar. Here’s a simple example:
// Layout.js
import React from ‘react’;
const Layout = ({ children }) => {
return (
<div>
<header>Header Content</header>
<aside>Sidebar Content</aside>
<main>{children}</main>
</div>
);
};
export default Layout;
Step 3: Define Your Routes
In your main app component, import `BrowserRouter,` `Routes,` and `Route` from `react-router-dom,` along with your layout and page components.
// App.js
import React from ‘react’;
import { BrowserRouter, Routes, Route } from ‘react-router-dom’;
import Layout from ‘./Layout’;
import HomePage from ‘./HomePage’;
import AboutPage from ‘./AboutPage’;
import OtherPage from ‘./OtherPage’;
const App = () => {
return (
<BrowserRouter>
<Routes>
<Route path=”/” element={<Layout />}>
<Route index element={<HomePage />} />
<Route path=”about” element={<AboutPage />} />
</Route>
<Route path=”other” element={<OtherPage />} />
</Routes>
</BrowserRouter>
);
};
export default App;
Step 4: Apply the Layout to Specific Routes
Step 5: Styling the Layout
You can add CSS to style your layout. Here’s a simple example using inline styles:
// Layout.js
const layoutStyle = {
display: ‘grid’,
gridTemplateAreas: `
‘header header’
‘sidebar main’
`,
gridTemplateColumns: ‘1fr 3fr’,
};
const headerStyle = {
gridArea: ‘header,’
};
const sidebarStyle = {
gridArea: ‘sidebar,’
};
const mainStyle = {
gridArea: ‘main,’
};
const Layout = ({ children }) => {
return (
<div style={layoutStyle}>
<header style={headerStyle}>Header Content</header>
<aside style={sidebarStyle}>Sidebar Content</aside>
<main style={mainStyle}>{children}</main>
</div>
);
};
Further Reading: Custom Web Application Development: A Complete Guide for C-Suite
Additional Tips:
- Use React Context: If your header or sidebar needs to be dynamic (e.g., changing based on user authentication status), consider using React Context to manage their states.
- Responsive Design: Consider making your layout responsive. You can use CSS media queries or a CSS framework like Bootstrap or Material-UI to help with this.
- Nested Routes: React Router DOM supports nested routes, which can be useful for creating complex layouts with multiple levels of navigation.
- Performance Optimization: For larger applications, consider code splitting using `React.lazy` and `Suspense` to load components only when needed, improving your app’s performance.
- Testing: Ensure to write tests for your routes and components. React Testing Library is a great tool for testing React components.
- Documentation: Always keep your code well-documented for ease of maintenance and clarity for other developers who might work on your project.
Need a Partner That Can Help You Enhance Your Web Application User Experience?
If you're struggling with Customer web development, we are here to help. Request a consultation and explore how to transform your app's interface for better navigation and style.
Request a ConsultationConclusion
If you followed all the guidelines in the blog, you must have now successfully created a layout component with a header and sidebar that applies to specific routes using the latest React Router DOM. This approach allows you to have different layouts for different application parts, making it versatile and adaptable to various design needs. Remember, you can always customize the layout, routes, and styles to suit your project’s requirements better.
As you become more comfortable with these concepts, you can explore more advanced features and customize your layout to better fit your project’s needs. Happy coding!
Explore Recent Blog Posts
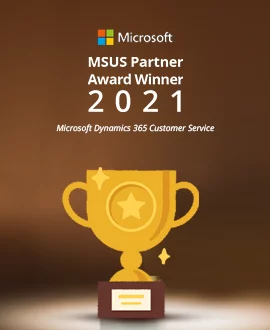